Search tool in your HUGO website.
As websites grow, incorporating a search feature becomes increasingly crucial. However, implementing search functionality can be challenging on a statically generated site since there’s no server-side component to handle the search. Instead, the search must be performed on the client side within the browser using JavaScript.
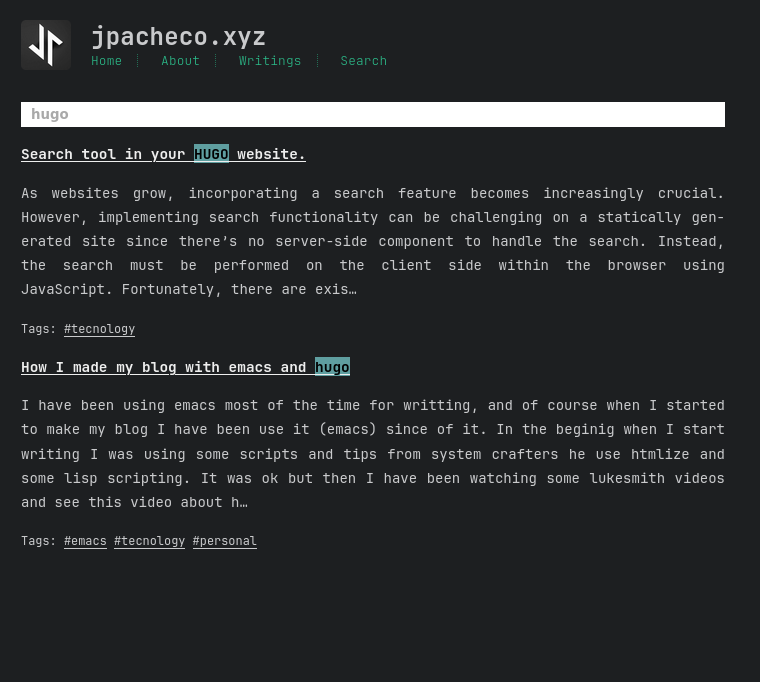
Figure 1: This is how my web looks.
Fortunately, there are existing solutions to address this issue. Below, we demonstrate one such solution that utilizes FuseJS and MarkJS. This implementation is inspired by a gist from eddiewebb, but it’s built using plain JavaScript rather than jQuery.
Page - content/search/_index.md
Create a page to serve as the search form and display search results. In the markdown, set the layout to ‘search’ and proceed to create this layout (in the next step) to easily manage the HTML.
1---
2title: "Search"
3sitemap:
4 priority : 0.1
5layout: "search"
6---
Layout - layout/_default/search.html
This search page contains a <div> element for displaying search results (#search-results) and another <div> element for showing a loading message (.search-loading). The layout also defines a template that will later be used in the JavaScript to render a single search result. Finally, it loads the necessary JS libraries (from a CDN in this example, though self-hosting is an option).
1{{ define "main" }}
2
3<main>
4 <form action="/search" method="GET">
5 <input type="search" name="q" id="search-query" placeholder="Search...." autofocus>
6 <!-- <button type="submit">Search</button> -->
7 </form>
8 <div id="search-results"></div>
9 <div class="search-loading">Loading...</div>
10
11 <script id="search-result-template" type="text/x-js-template">
12 <div id="summary-${key}">
13 <h3><a href="${link}">${title}</a></h3>
14 <p>${snippet}</p>
15 <p>
16 <small>
17 ${ isset tags }Tags: ${tags}<br>${ end }
18 </small>
19 </p>
20 </div>
21 </script>
22
23 <script src="https://cdnjs.cloudflare.com/ajax/libs/fuse.js/6.6.2/fuse.min.js" integrity="sha512-Nqw1tH3mpavka9cQCc5zWWEZNfIPdOYyQFjlV1NvflEtQ0/XI6ZQ+H/D3YgJdqSUJlMLAPRj/oXlaHCFbFCjoQ==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
24
25 <script src="https://cdnjs.cloudflare.com/ajax/libs/mark.js/8.11.1/mark.min.js" integrity="sha512-5CYOlHXGh6QpOFA/TeTylKLWfB3ftPsde7AnmhuitiTX4K5SqCLBeKro6sPS8ilsz1Q4NRx3v8Ko2IBiszzdww==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
26</main>
27
28{{ end }}
JS
The main part of the code is JavaScript, where everything is tied together. The URL parameters are read to obtain the query, which is then passed to FuseJS and MarkJS, and the results are written back to the page.
1var summaryInclude = 180;
2var fuseOptions = {
3 shouldSort: true,
4 includeMatches: true,
5 includeScore: true,
6 tokenize: true,
7 location: 0,
8 distance: 100,
9 minMatchCharLength: 1,
10 keys: [
11 {name: "title", weight: 0.45},
12 {name: "contents", weight: 0.4},
13 {name: "tags", weight: 0.1},
14 {name: "categories", weight: 0.05}
15 ]
16};
17
18// =============================
19// Search
20// =============================
21
22var inputBox = document.getElementById('search-query');
23if (inputBox !== null) {
24 var searchQuery = param("q");
25 if (searchQuery) {
26 inputBox.value = searchQuery || "";
27 executeSearch(searchQuery, false);
28 } else {
29 document.getElementById('search-results').innerHTML = '<p class="search-results-empty">Please enter a word or phrase above, or see <a href="/tags/">all tags</a>.</p>';
30 }
31}
32
33function executeSearch(searchQuery) {
34
35 show(document.querySelector('.search-loading'));
36
37 fetch('/index.json').then(function (response) {
38 if (response.status !== 200) {
39 console.log('Looks like there was a problem. Status Code: ' + response.status);
40 return;
41 }
42 // Examine the text in the response
43 response.json().then(function (pages) {
44 var fuse = new Fuse(pages, fuseOptions);
45 var result = fuse.search(searchQuery);
46 if (result.length > 0) {
47 populateResults(result);
48 } else {
49 document.getElementById('search-results').innerHTML = '<p class=\"search-results-empty\">No matches found</p>';
50 }
51 hide(document.querySelector('.search-loading'));
52 })
53 .catch(function (err) {
54 console.log('Fetch Error :-S', err);
55 });
56 });
57}
58
59function populateResults(results) {
60
61 var searchQuery = document.getElementById("search-query").value;
62 var searchResults = document.getElementById("search-results");
63
64 // pull template from hugo template definition
65 var templateDefinition = document.getElementById("search-result-template").innerHTML;
66
67 results.forEach(function (value, key) {
68
69 var contents = value.item.contents;
70 var snippet = "";
71 var snippetHighlights = [];
72
73 snippetHighlights.push(searchQuery);
74 snippet = contents.substring(0, summaryInclude * 2) + '…';
75
76 //replace values
77 var tags = ""
78 if (value.item.tags) {
79 value.item.tags.forEach(function (element) {
80 tags = tags + "<a href='/tags/" + element + "'>" + "#" + element + "</a> "
81 });
82 }
83
84 var output = render(templateDefinition, {
85 key: key,
86 title: value.item.title,
87 link: value.item.permalink,
88 tags: tags,
89 categories: value.item.categories,
90 snippet: snippet
91 });
92 searchResults.innerHTML += output;
93
94 snippetHighlights.forEach(function (snipvalue, snipkey) {
95 var instance = new Mark(document.getElementById('summary-' + key));
96 instance.mark(snipvalue);
97 });
98
99 });
100}
101
102function render(templateString, data) {
103 var conditionalMatches, conditionalPattern, copy;
104 conditionalPattern = /\$\{\s*isset ([a-zA-Z]*) \s*\}(.*)\$\{\s*end\s*}/g;
105 //since loop below depends on re.lastInxdex, we use a copy to capture any manipulations whilst inside the loop
106 copy = templateString;
107 while ((conditionalMatches = conditionalPattern.exec(templateString)) !== null) {
108 if (data[conditionalMatches[1]]) {
109 //valid key, remove conditionals, leave contents.
110 copy = copy.replace(conditionalMatches[0], conditionalMatches[2]);
111 } else {
112 //not valid, remove entire section
113 copy = copy.replace(conditionalMatches[0], '');
114 }
115 }
116 templateString = copy;
117 //now any conditionals removed we can do simple substitution
118 var key, find, re;
119 for (key in data) {
120 find = '\\$\\{\\s*' + key + '\\s*\\}';
121 re = new RegExp(find, 'g');
122 templateString = templateString.replace(re, data[key]);
123 }
124 return templateString;
125}
126
127// Helper Functions
128function show(elem) {
129 elem.style.display = 'block';
130}
131function hide(elem) {
132 elem.style.display = 'none';
133}
134function param(name) {
135 return decodeURIComponent((location.search.split(name + '=')[1] || '').split('&')[0]).replace(/\+/g, ' ');
136}
137
138// Keybinds functions
139document.addEventListener('keydown', function(event) {
140 // Check if the Windows key (or Command key on macOS) and forward slash are pressed
141 if (event.metaKey && event.key === '/') {
142 // Prevent the default action if necessary
143 event.preventDefault();
144
145 // Navigate to the search page
146 window.location.href = '/search';
147
148 // Wait for the page to load and then focus on the search input
149 window.addEventListener('load', function() {
150 const searchInput = document.getElementById('search-query');
151 if (searchInput) {
152 searchInput.focus();
153 }
154 });
155 }
156});
The Data - layouts/_default/index.json
All the work we’ve done up until now has been front-end workings, but we also need to build an index.json file which will act as our data source (which is fetched from the JS).
1{{- $.Scratch.Add "index" slice -}}
2{{- range .Site.RegularPages -}}
3 {{- $.Scratch.Add "index" (dict "title" .Title "tags" .Params.tags "categories" .Params.categories "contents" .Plain "permalink" .Permalink) -}}
4{{- end -}}
5{{- $.Scratch.Get "index" | jsonify -}}
Conclusion
You can extend this further by offering a live search preview, adding more filters, or allowing sorting options. This method provides a powerful and flexible way to implement search functionality on a static site without relying on any backend services.